Improving Performance and Scalability with Node.js Clustering
Learn how to improve the performance and reliability of your Node.js applications with clustering. Discover the benefits of clustering, how to implement it, and best practices for optimizing worker performance, handling memory leaks, and avoiding common pitfalls.
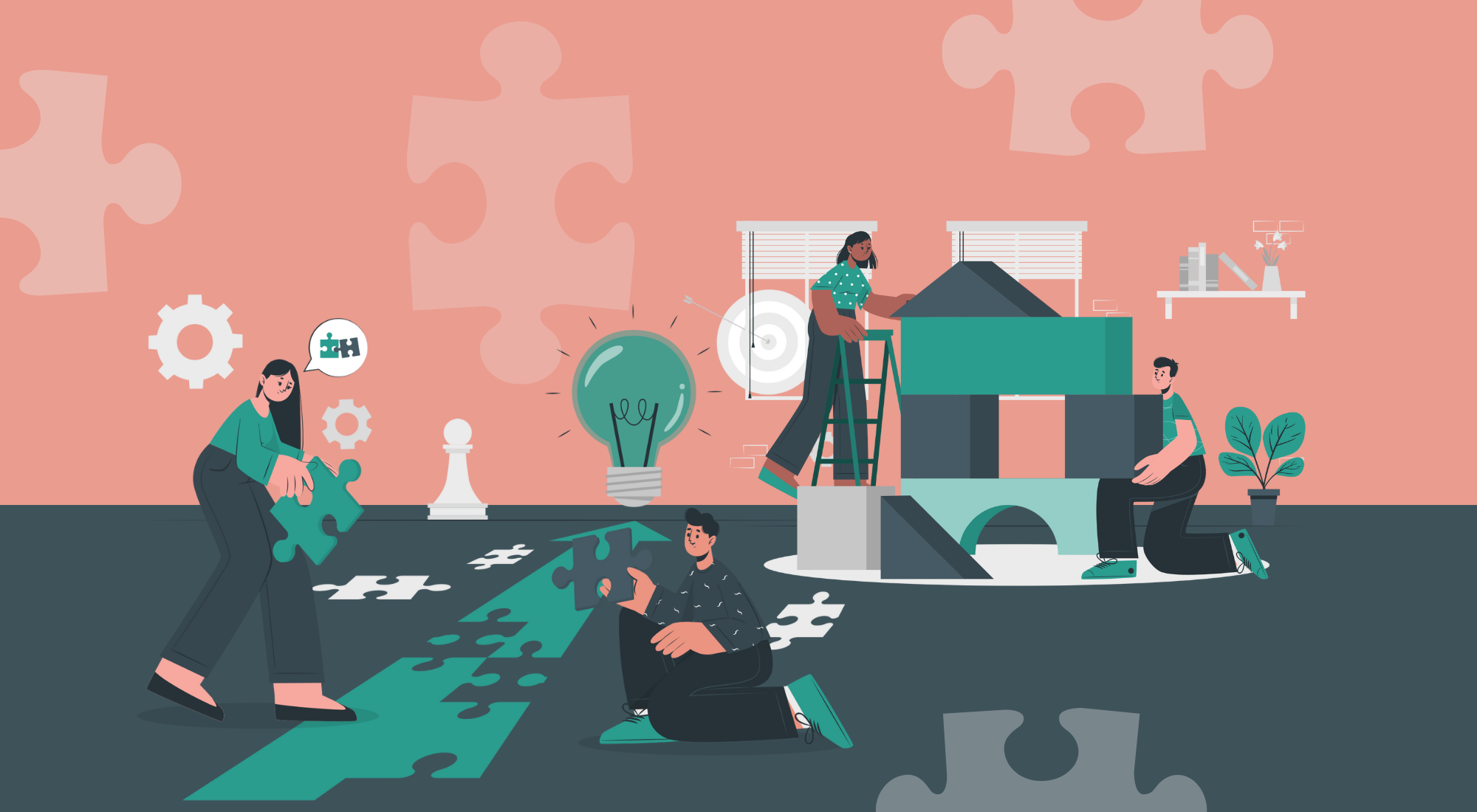
Introduction
Have you ever been to a restaurant that was so popular, it felt like you were waiting in line forever just to get a table? Or maybe you've visited a website that was so slow, it felt like you were watching paint dry while waiting for the page to load. If you've experienced these frustrations, then you know the importance of performance and scalability in both the physical and digital worlds.
In the world of web development, performance and scalability are key factors in the success of any application. As your user base grows, your application needs to be able to handle the increased traffic and demand without slowing down or crashing. That's where Clustering comes in.
In this article, we'll explore the world of clustering in Node.js, and how it can help you improve the performance and scalability of your web applications. We'll cover the basics of clustering, how to enable clustering in a Node.js application, load balancing with clustering, and best practices for optimizing performance and reliability. So let's dive in and learn how to build high-performance web applications with Node.js clustering!
Understanding Clustering
Clustering is like having a team of chefs in a busy restaurant kitchen. Just as a single chef can only prepare a limited number of dishes at once, a single Node.js process can only handle a limited number of requests at once. By enabling clustering, you can create a team of Node.js processes that work together to handle a much larger volume of requests, just like a team of chefs working together can prepare a larger volume of dishes.
For simpler cases, Node.js applications can make use of the cluster
module to implement Clustering, which provides an easy-to-use API for creating worker processes and managing communication between them. There are two types of workers in a clustered Node.js application: the master process and the worker processes. The master process is responsible for creating and managing the worker processes, as well as handling communication between them. The worker processes are responsible for handling incoming requests, running user code, and returning responses to the client.
The master process is created automatically when a Node.js application is started in cluster mode, using the cluster.fork()
method to create one or more worker processes. Each worker process runs a copy of the application code, but they operate independently of each other, handling requests in parallel. The master process acts as a supervisor, monitoring the health of the worker processes, restarting them if they crash, and managing the distribution of incoming requests among them.
To communicate with each other, the worker processes use inter-process communication (IPC) channels provided by the cluster
module. This allows them to share data and resources, such as database connections or caches, and coordinate their activities. The master process can also communicate with the worker processes using IPC, for example to send commands to shut down or restart the application.
In summary, clustering in Node.js involves creating multiple worker processes to handle incoming requests in parallel, improving the performance and scalability of the application. The cluster module provides an easy-to-use API for creating and managing these worker processes, with the master process acting as a supervisor and handling communication between them. In the next section, we'll look at how to enable clustering in a Node.js application.
Clustering in practice
Before we get started, let's set up a simple Node.js project. We'll be using the popular Express framework to create a basic web server with a single route that performs a CPU-bound computation.
Getting Started
The first step is to ensure that you have Node.js and NPM (Node.js Package Manager) installed on your system. If you haven't already done so, you can download and install Node.js from the official website. Once you've installed Node.js, npm should also be installed automatically.
The next step is to create a new Node.js project and installing dependencies. You can do this by creating a new directory and running the following commands:
npm init -y
npm install express
With our project set up, let's create a new file called index.js
and add the following code:
const express = require('express');
const app = express();
console.log(`Worker ${process.pid} started`);
app.get('/', (req, res) => {
// Perform a CPU-bound computation
const result = fibonacci(40);
res.send(`Result: ${result}`);
});
function fibonacci(n) {
if (n <= 1) {
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
app.listen(3000, () => {
console.log('Server running on port 3000');
});
This code sets up a basic Express web server with a single route that calculates the 40th number in the Fibonacci sequence, a CPU-bound computation that takes some time to complete.
Save the changes to index.js
and start the server using the following command in your terminal:
node index.js
Here is what the console output should look like:
Worker 36904 started
Server running on port 3000
At this point, you have a simple Node.js app running on http://localhost:3000
with a single route that performs a CPU-bound computation and returns the result.
With the server running, test your route by running the following command in a new terminal tab:
curl http://localhost:3000/
Here is what the console output should look like:
Result: 102334155
The output confirms that the route we created is working as expected.
Enable Clustering
With our server set up, let's move on to enabling clustering. As mentioned earlier, we'll be using the built-in cluster
module that comes with Node.js to do this. This module also comes with a load-balancer that distributes load in a round-robin fashion.
Create a new file called cluster.js
in the same directory as index.js
.
touch cluster.js
Now add the following snippet of code to cluster.js
:
const cluster = require('cluster');
const os = require('os');
if (cluster.isMaster) {
const numWorkers = os.cpus().length;
console.log(`Master process is running with PID ${process.pid} and creating ${numWorkers} worker processes.`);
for (let i = 0; i < numWorkers; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker process with PID ${worker.process.pid} exited with code ${code} and signal ${signal}.`);
console.log(`Starting a new worker process...`);
cluster.fork();
});
} else {
require('./index.js');
}
First, we import the cluster
module and the built-in os
module. Then we check if the current process is the master process using cluster.isMaster
. If it is, we get the number of available CPU cores using os.cpus().length
. We then log a message indicating that the master process is running and creating worker processes.
We then use a for
loop to create a worker process for each CPU core using cluster.fork()
. This creates a separate Node.js process for each core that will handle incoming requests.
We also listen for the exit
event on the cluster object using cluster.on('exit', ...)
. This event is emitted when a worker process dies for any reason. When this happens, we log a message indicating which worker process died and start a new worker process using cluster.fork()
.
If the current process is not the master process, then it must be a worker process. In this case, we simply require the index.js
file, which contains the actual application logic that we want to run in each worker process.
So in summary, the cluster.js
file creates a separate Node.js process for each CPU core and runs the application logic in each process using the index.js
file. It also automatically restarts any worker processes that die for any reason.
Now if you run:
node cluster.js
Here is what the output will look like:
Worker 37005 started
Worker 37007 started
Server running on port 3000
Worker 37010 started
Worker 37012 started
Worker 37011 started
Server running on port 3000
Worker 37003 started
Worker 37004 started
Worker 37014 started
Worker 37009 started
Worker 37008 started
Worker 37006 started
Server running on port 3000
Server running on port 3000
Server running on port 3000
Worker 37013 started
Server running on port 3000
Server running on port 3000
Server running on port 3000
Server running on port 3000
Server running on port 3000
Server running on port 3000
Server running on port 3000
This spawned a total of 12 workers in my case.
Let's go ahead and test the route we created earlier with clustering enabled:
curl http://localhost:3000/
The output confirms that the application is working as expected:
Result: 102334155
Performance Comparison
Now that we have enabled clustering in our Node.js application, we can compare the performance of the application with and without clustering using a load testing tool. Load testing allows us to simulate high traffic conditions and measure the response time and throughput of the application under different levels of load.
To perform load testing, we will use the ab
(Apache Bench) command-line tool, which is included in most Apache installations. ab
allows us to send a specified number of requests to the server and measure the average response time and throughput. Other options include the loadtest
npm package.
Without Clustering
Let's run our application without clustering enabled:
node index.js
In another terminal tab run the following command:
ab -n 500 -c 50 http://localhost:3000/
This command sends 500 requests (-n 500
) with a concurrency of 50 (-c 50
) to the URL http://localhost:3000/
.
The results include the average response time, throughput and other useful metrics:
Benchmarking localhost (be patient)
Completed 100 requests
Completed 200 requests
Completed 300 requests
Completed 400 requests
Completed 500 requests
Finished 500 requests
Server Software:
Server Hostname: localhost
Server Port: 3000
Document Path: /
Document Length: 17 bytes
Concurrency Level: 50
Time taken for tests: 331.365 seconds
Complete requests: 500
Failed requests: 0
Total transferred: 108500 bytes
HTML transferred: 8500 bytes
Requests per second: 1.51 [#/sec] (mean)
Time per request: 33136.491 [ms] (mean)
Time per request: 662.730 [ms] (mean, across all concurrent requests)
Transfer rate: 0.32 [Kbytes/sec] received
Connection Times (ms)
min mean[+/-sd] median max
Connect: 0 2 0.6 2 3
Processing: 686 32123 4697.6 33167 36448
Waiting: 660 24399 6134.5 25052 33274
Total: 686 32125 4697.8 33169 36450
Percentage of the requests served within a certain time (ms)
50% 33169
66% 33197
75% 33224
80% 33253
90% 33826
95% 34414
98% 36449
99% 36449
100% 36450 (longest request)
With Clustering
Now let's run our application with clustering enabled:
node cluster.js
In another terminal tab run the following command:
ab -n 500 -c 50 http://localhost:3000/
Once again this command sends 500 requests (-n 500
) with a concurrency of 50 (-c 50
) to the URL http://localhost:3000/
.
Here are the results:
Benchmarking localhost (be patient)
Completed 100 requests
Completed 200 requests
Completed 300 requests
Completed 400 requests
Completed 500 requests
Finished 500 requests
Server Software:
Server Hostname: localhost
Server Port: 3000
Document Path: /
Document Length: 17 bytes
Concurrency Level: 50
Time taken for tests: 38.240 seconds
Complete requests: 500
Failed requests: 0
Total transferred: 108500 bytes
HTML transferred: 8500 bytes
Requests per second: 13.08 [#/sec] (mean)
Time per request: 3823.983 [ms] (mean)
Time per request: 76.480 [ms] (mean, across all concurrent requests)
Transfer rate: 2.77 [Kbytes/sec] received
Connection Times (ms)
min mean[+/-sd] median max
Connect: 0 2 2.2 0 12
Processing: 676 3588 620.6 3732 4501
Waiting: 675 3587 620.7 3732 4501
Total: 677 3589 620.6 3734 4503
Percentage of the requests served within a certain time (ms)
50% 3734
66% 3811
75% 3862
80% 3889
90% 4043
95% 4125
98% 4298
99% 4322
100% 4503 (longest request)
Analysis
Without Clustering:
- Requests per second: 1.51 [#/sec] (mean)
- Time per request: 33136.491 [ms] (mean)
- Time per request: 662.730 [ms] (mean, across all concurrent requests)
With Clustering:
- Requests per second: 13.08 [#/sec] (mean)
- Time per request: 3823.983 [ms] (mean)
- Time per request: 76.480 [ms] (mean, across all concurrent requests)
From the results, it's clear that the clustered version of the application performed significantly better than the non-clustered version. The requests per second metric is an indicator of how many requests the server can handle per second, and it's clear that the clustered version can handle more than eight times as many requests per second as the non-clustered version.
Similarly, the time per request metric shows that requests are handled much more quickly by the clustered version. The clustered version is more than eight times faster than the non-clustered version on average, which means that users will experience much faster response times when using the clustered version.
Overall, the load testing results clearly demonstrate the benefits of using clustering to scale Node.js applications. By taking advantage of all available CPU cores, clustering can significantly improve the performance and scalability of Node.js applications, enabling them to handle much higher loads and respond more quickly to user requests.
Best Practices
Clustering is a powerful feature of Node.js that allows you to take full advantage of multi-core processors and increase the performance and scalability of your applications. However, as with any powerful tool, it's important to use it correctly to avoid common pitfalls and optimize worker performance.
Here are some best practices to keep in mind when implementing clustering in Node.js applications:
- Optimize worker performance: Each worker process in a Node.js cluster is an independent instance of your application, so it's important to optimize their performance. This can include techniques like using a lightweight framework, avoiding synchronous I/O calls, and minimizing memory usage. By doing this, you can ensure that each worker process is able to handle the maximum number of requests and provide a smooth and responsive experience to your users.
- Use Sticky Sessions: To avoid the overhead of inter-process communication (IPC) and ensure that requests from the same client are routed to the same worker process, use sticky sessions. Sticky sessions ensure that the client's session is always handled by the same worker process, improving performance and reducing the likelihood of errors.
- Handle memory leaks: Memory leaks can be a major problem in clustered Node.js applications, as they can quickly consume available system resources and cause performance issues. To avoid memory leaks, you should regularly monitor your application's memory usage and use tools like heap snapshots to identify potential leaks. Additionally, you should make sure to dispose of any unnecessary resources and release memory when they are no longer needed.
- Use a process manager: While the Node.js
cluster
module provides a simple way to manage worker processes, it is often not sufficient for more complex applications. In these cases, you may want to consider using a process manager like PM2 instead, which provides additional features like automatic restarts, logging, and monitoring. - Monitor system resources: It's important to regularly monitor system resources like CPU usage, memory usage, and network activity to ensure that your application is running smoothly and not consuming too many resources. This can help you identify potential performance issues and take corrective action before they become critical.
By following these best practices, you can ensure that your clustered Node.js applications are fast, responsive, and reliable, and provide the best possible experience to your users.
Conclusion
In conclusion, clustering is a powerful tool for optimizing the performance and scalability of Node.js applications. By distributing the workload across multiple CPU cores, clustering can significantly improve the response time and throughput of your application, making it capable of handling a large number of concurrent requests. However, it's important to follow best practices when implementing clustering, such as optimizing worker performance, handling memory leaks, and using sticky sessions when necessary. You can find the complete code used in this article here.
At Sych, we specialize in building custom software solutions for businesses that require high performance and scalability. Our team of experienced developers can help you optimize your Node.js applications using clustering and other advanced techniques. Contact us today to learn more about how we can help your business achieve its goals.