From Good to Great: Advanced ReactJS Techniques for Taking Your Applications to the Next Level
Learn advanced ReactJS techniques such as server-side rendering, lazy loading, performance optimization, handling large datasets, and error handling to build fast, stable, and user-friendly applications.
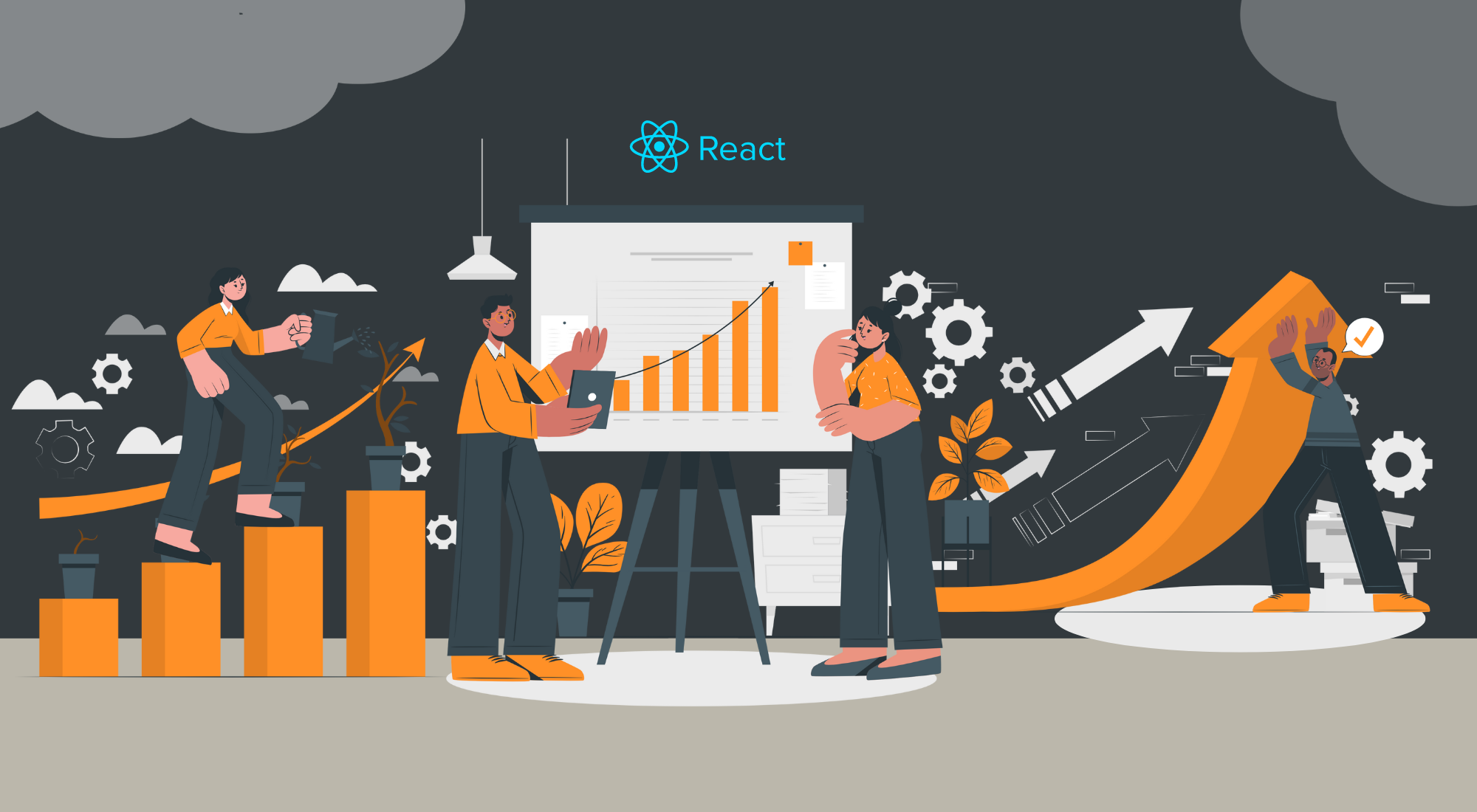
Introduction
Do you ever feel like you've hit a ceiling with ReactJS? You've built some basic applications and feel comfortable with the basics, but you're eager to take your skills to the next level. If so, you're not alone! ReactJS is a powerful and versatile framework, and there are many advanced techniques that you can use to make your applications faster, more efficient, and more user-friendly.
As a developer, I've faced these challenges myself. After building several ReactJS applications, I found myself wanting to dive deeper and explore more complex topics. Through my research and experience, I've discovered a range of tools and strategies that have helped me take applications I build to the next level.
Whether you're building an application from scratch or looking to optimize an existing one, the topics I cover in this article will help you improve performance, handle large datasets, and deliver better UX. Let's dive in!
Lazy Loading
Have you ever visited a website that takes forever to load, or noticed that your own ReactJS applications are slow to start up? If so, lazy loading may be the solution you're looking for.
Lazy Loading is a technique that allows you to defer the loading of non-critical parts of your application until they are needed. This can improve the performance of your application by reducing the amount of code that needs to be loaded initially, which can lead to faster load times and a better user experience.
I've found that lazy loading can be particularly useful for applications that have a lot of large assets, such as images or videos. By only loading these assets when they're actually needed, you can reduce the amount of data that needs to be loaded upfront and improve the perceived speed of your application.
In React, you can implement Lazy Loading using the React.lazy()
function and the Suspense
component. Here's an example of how you might use Lazy Loading to defer the loading of a component:
import React, { lazy, Suspense } from 'react';
const MyLazyComponent = lazy(() => import('./MyLazyComponent'));
function MyComponent() {
return (
<div>
<p>This is the main content of the page.</p>
<Suspense fallback={<div>Loading...</div>}>
<MyLazyComponent />
</Suspense>
</div>
);
}
export default MyComponent;
In this example, we've used the React.lazy()
function to lazily load our MyLazyComponent
component. We've also wrapped our MyLazyComponent
component with a Suspense
component, which allows us to provide a loading state while our component is being loaded. Note that the fallback
prop of the Suspense
component is required, and it should be a fallback UI that is displayed while the lazy-loaded component is being loaded.
Lazy Loading can be a powerful technique for improving the performance of your React application, but it's important to use it judiciously. Be sure to only lazy load components that are non-critical or that are not needed immediately, and avoid overusing Lazy Loading as it can add unnecessary complexity to your code.
Server-side Rendering
Have you ever noticed that your ReactJS applications take a long time to load, or that search engines don't index them properly? If so, server-side rendering (SSR) may be the solution you're looking for.
SSR is a technique that allows you to render your ReactJS application on the server before sending it to the client's browser. This can lead to faster loading times, better search engine optimization (SEO), and improved overall performance for your application.
As a developer, I've found that SSR can be particularly useful for applications that require a lot of data or have a large number of routes. By rendering the initial view on the server, you can reduce the amount of data that needs to be loaded on the client side and improve the perceived speed of your application.
To implement SSR in a ReactJS application, you can use frameworks like Next.js or Gatsby, which have built-in support for SSR. Alternatively, you can build your own server-side rendering solution using libraries like ReactDOMServer.
One thing to keep in mind when using SSR is that it can add complexity to your application, particularly if you're not familiar with server-side programming. You'll need to think about things like data fetching, routing, and server-side caching in order to make sure your application performs well.
Overall, however, I've found that the benefits of SSR are well worth the effort. By implementing SSR in your ReactJS application, you can improve performance, SEO, and overall user experience.
Performance Optimization
If you're building a web application with React, you want it to be fast and responsive. After all, who wants to use a sluggish website that takes forever to load? Luckily, React provides a variety of built-in tools and techniques that can help you optimize the performance of your application and ensure that it runs smoothly and efficiently.
Minimize Re-renders
One of the keys to optimizing performance in React is to minimize the number of re-renders that occur when your application state changes. This might sound like a daunting task, but React makes it easy with tools like memo
, useMemo
, useCallback
, shouldComponentUpdate
and PureComponent
which help you memoize components, expensive computations, and event handlers. By minimizing unnecessary re-renders, you can keep your application running smoothly and efficiently.
Here's an example of using memo
to memoize components:
import { memo } from 'react';
const MyComponent = memo(({ prop1, prop2 }) => {
return (
<div>
<h1>{prop1}</h1>
<p>{prop2}</p>
</div>
);
});
In this example, the MyComponent
component will only re-render if its prop1
or prop2
props change. By using memo
, we can ensure that our component only re-renders when necessary, which can help improve performance.
Here's another example which uses shouldComponentUpdate
to prevent unnecessary re-renders:
import React from 'react';
class MyComponent extends React.Component {
shouldComponentUpdate(nextProps, nextState) {
// Only re-render if the `value` prop has changed
return nextProps.value !== this.props.value;
}
render() {
const { value } = this.props;
return <p>{value}</p>;
}
}
In this example, the MyComponent
component will only re-render if its value
prop has changed. By using the shouldComponentUpdate
method, we can prevent unnecessary re-renders and help improve performance.
Minimize Application Bundle Size
Another important technique for optimizing performance in React is to minimize the size of your application's JavaScript bundle. This involves using techniques like Code Splitting (already discussed under Lazy-Loading) and Tree Shaking which is removal of unused code from your bundle, significantly reducing its size. You can use tools like Webpack and Rollup to perform Tree Shaking. By minimizing the size of your application's JavaScript bundle, you can ensure that it loads quickly and efficiently, even on slower network connections.
Use Profiling Tools
You can also use performance profiling tools like the React Profiler API or third-party tools like React Developer Tools or Reactotron to identify performance bottlenecks in your application and optimize your code accordingly.
Optimize for the Browser
It is also important to optimize your React application for the browser environment in which it will be running. This might involve using browser-specific optimizations like requestAnimationFrame
to schedule updates instead of setInterval
or setTimeout
, or using CSS transitions and animations instead of JavaScript animations to minimize the amount of work the browser needs to do. requestAnimationFrame
is part of the browser API that allows you to schedule animations and other updates to occur during the next frame repaint. By using requestAnimationFrame
, you can avoid causing unnecessary repaints and improve the smoothness of your animations.
Here's an example of using requestAnimationFrame()
to schedule updates:
import React, { useState, useRef } from 'react';
const MyComponent = () => {
const [value, setValue] = useState(0);
const lastUpdateRef = useRef(0);
const updateValue = () => {
// Only update the value every 16ms (60fps)
const now = performance.now();
if (now - lastUpdateRef.current > 16) {
lastUpdateRef.current = now;
setValue(value => value + 1);
}
requestAnimationFrame(updateValue);
};
// Start the animation loop when the component mounts
useRef(updateValue)();
return <p>{value}</p>;
};
In this example, the MyComponent
component updates its value
state using requestAnimationFrame
to schedule updates every 16ms. By using requestAnimationFrame
, we can ensure that our updates are synchronized with the browser's rendering pipeline, which can help minimize jank and improve performance.
By using these techniques and others like them, you can create high-performance web applications that provide a great user experience and can handle even the most demanding workloads. So why not give them a try and see how they can help you take your React applications to the next level?
Handling Large Datasets
If you've ever worked with large datasets in a ReactJS application, you know how challenging it can be to maintain performance and responsiveness while dealing with all that data. Fortunately, there are techniques and tools you can use to help you handle large datasets in your ReactJS application.
Apart from using server-side filtering or use of useMemo
and useCallback
hooks (discussed earlier), one common approach is to use pagination or infinite scrolling to load data in smaller, more manageable chunks. This can help reduce the initial load time of your application and improve overall performance. You can use libraries like React Virtualized or React Window to efficiently render large lists or tables by only rendering the visible portion of the data at any given time.
Here's an example of how you can use react-window
to render a list of items efficiently:
import React, { useState } from "react";
import { FixedSizeList } from "react-window";
const Row = ({ index, style, data }) => (
<div style={style}>
{data[index]}
</div>
);
const MyList = () => {
const [items, setItems] = useState(Array.from({ length: 10000 }, (_, i) => `Item ${i}`));
return (
<FixedSizeList height={400} width={400} itemSize={35} itemCount={items.length} itemData={items}>
{Row}
</FixedSizeList>
);
};
export default MyList;
In this example, we're using FixedSizeList
from react-window
to render a list of 10,000 items. The FixedSizeList
component only renders the items that are currently visible in the viewport, rather than rendering all 10,000 items at once. The Row
component is used to render each individual item in the list. We pass this component to FixedSizeList
as a child, along with the height
, width
, itemSize
, itemCount
, and itemData
props.
Error Handling
As a ReactJS developer, you know that things don't always go as planned. Errors can occur for a variety of reasons, whether it's a network failure, user input error, or an unexpected bug in your code. However, with the right error handling techniques, you can ensure that your ReactJS application remains stable and user-friendly, even in the face of errors.
Error Boundaries
One of the common techniques is use of an ErrorBoundary
component. By default, if your application throws an error during rendering, React will remove the UI from the screen. To prevent this, you can wrap the problematic part of your UI in an ErrorBoundary
component, which will display a fallback UI instead of the crashed UI. Here's an example:
import React, { Component } from 'react';
class ErrorBoundary extends Component {
state = {
hasError: false
}
static getDerivedStateFromError(error) {
// Update state so that the next render will show the fallback UI.
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// Log the error to an error reporting service
logErrorToMyService(error, errorInfo);
}
render() {
if (this.state.hasError) {
// Fallback UI if an error occurs
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
export default ErrorBoundary;
In this example, the ErrorBoundary
component has two lifecycle methods: getDerivedStateFromError
and componentDidCatch
. getDerivedStateFromError
is called when an error occurs and updates the component's state to display the fallback UI. componentDidCatch
is called after the error has been caught and can be used to log the error to a service, such as an analytics service.
To use an ErrorBoundary
, simply wrap the component or components that you want to handle errors for with the ErrorBoundary
component, like so:
import React from 'react';
import ErrorBoundary from './ErrorBoundary';
function App() {
return (
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
);
}
In this example, any errors that occur within the MyComponent
component will be caught by the ErrorBoundary
and a fallback UI will be displayed instead of crashing the entire application.
Use try/catch
Another good practice for handling errors in React is to use the try/catch
statements to catch errors that occur within a specific block of code. For example:
function myFunction() {
try {
// Code that may throw an error
} catch (error) {
// Code to handle the error
}
}
In this example, any errors that occur within the try
block will be caught by the catch
statement, allowing you to handle the error in a specific way.
Use Error Tracking Tools
Apart from logging and displaying informative error messages to the user, which can help users understand what went wrong, it's also important to consider how you're logging errors in your ReactJS application. By using tools like Sentry or Rollbar, you can track errors and performance issues in real-time and receive notifications when errors occur. This can help you identify and resolve issues quickly, before they have a significant impact on your users.
Investing time and effort into error handling can have a big impact on the success of your ReactJS application. By ensuring that your application remains stable and user-friendly, even in the face of errors, you can improve user experience, reduce bounce rates, and ensure that your application is scalable and efficient, even as your user base grows.
Conclusion
In conclusion, mastering ReactJS is no small feat, but it's definitely achievable with the right mindset, skills, and tools. As the ReactJS ecosystem continues to evolve and grow, there will be new challenges and opportunities to explore. However, by staying curious, keeping an open mind, and continually seeking out new knowledge and skills, you can stay ahead of the curve and continue to create amazing, high-performance applications that delight your users.
If you're looking for a team of experienced developers to help you build software that's tailored to your business's specific needs and goals, consider reaching out to Sych. With our deep expertise in ReactJS development, as well as other cutting-edge technologies and methodologies, we can help you bring your vision to life and create scalable applications that delivers real value to your customers and stakeholders. So why wait? Contact us today to learn more about our services and how we can help you achieve your business goals.